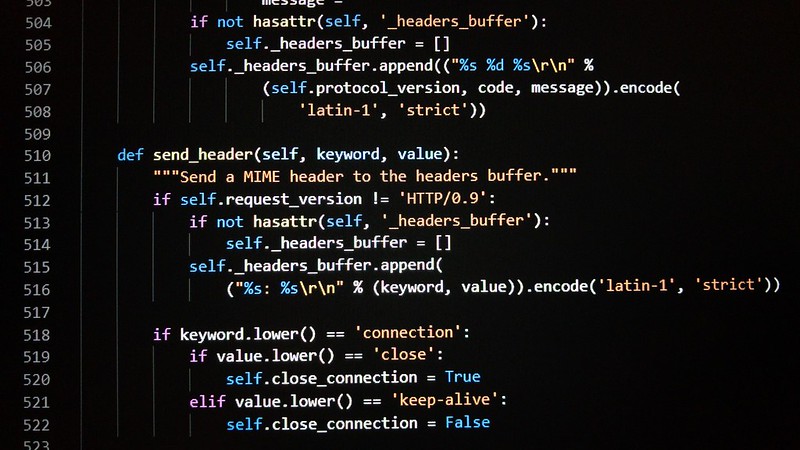
Output Formatting Options
Python provides multiple ways to format output for display or writing to files. The simplest methods are expression statements and the print()
function, but for more sophisticated formatting, you have several options:
Formatted String Literals (f-strings)
Introduced in Python 3.6, f-strings provide a concise way to embed expressions inside string literals:
year = 2016
event = 'Referendum'
print(f'Results of the {year} {event}')
# Output: Results of the 2016 Referendum
F-strings support formatting specifications after expressions:
import math
print(f'Pi value: {math.pi:.3f}') # Rounds to 3 decimal places
For aligning columns:
table = {'Sjoerd': 4127, 'Jack': 4098, 'Dcab': 7678}
for name, phone in table.items():
print(f'{name:10} ==> {phone:10d}')
The str.format() Method
This method provides more explicit control over formatting:
print('We are the {} who say "{}!"'.format('knights', 'Ni'))
You can reference values by position or keyword:
print('{0} and {1}'.format('spam', 'eggs'))
print('This {food} is {adj}.'.format(food='spam', adj='horrible'))
Manual String Formatting
For complete control, you can use string methods:
for x in range(1, 11):
print(str(x).rjust(2), str(x*x).rjust(3), str(x*x*x).rjust(4))
String methods include:
str.rjust(width)
– Right justifystr.ljust(width)
– Left justifystr.center(width)
– Centerstr.zfill(width)
– Pad with zeros
String Representation
For debugging, use repr()
and str()
to convert values to strings:
s = 'Hello, world.'
print(str(s)) # Human-readable
print(repr(s)) # Interpreter-readable
Working with Files
Opening Files
Use the open()
function with recommended encoding:
with open('workfile', 'r', encoding='utf-8') as f:
contents = f.read()
File modes include:
'r'
– Read (default)'w'
– Write (overwrites)'a'
– Append'r+'
– Read and write'b'
– Binary mode
File Object Methods
Key methods for reading:
f.read(size)
– Reads specified amountf.readline()
– Reads single linef.readlines()
– Returns list of lines- Iteration – Memory efficient line reading
Writing methods:
f.write(string)
– Writes string to filef.tell()
– Returns current positionf.seek(offset)
– Changes file position
JSON Data Handling
For structured data, use the json
module:
import json
# Serialize
data = {'name': 'John', 'age': 30}
with open('data.json', 'w') as f:
json.dump(data, f)
# Deserialize
with open('data.json', 'r') as f:
loaded = json.load(f)
Note: JSON files must use UTF-8 encoding.
Best Practices
- Always use
with
statements for file handling to ensure proper closing - Specify encoding (preferably UTF-8) when opening text files
- Use binary mode (
'b'
) for non-text files - Prefer f-strings for most string formatting needs
- Use JSON for structured data storage
- Consider
repr()
for debugging output to see exact values
Remember that file paths are system-dependent – use raw strings (r'path'
) or forward slashes for Windows paths to avoid escape character issues.