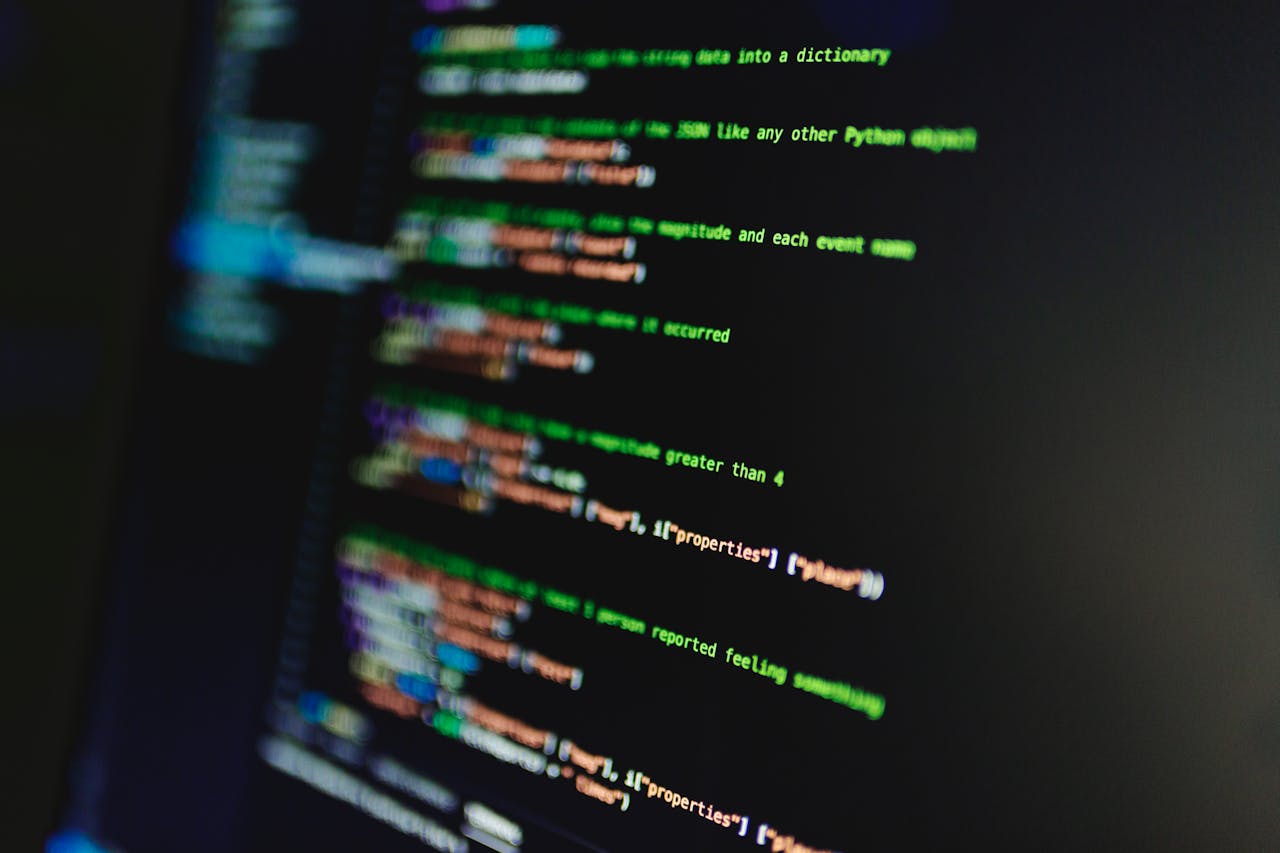
Python offers several control flow statements beyond the basic while
loop that provide flexibility in how you structure your programs.
Conditional Statements with if
The if
statement is one of Python’s most fundamental control structures:
x = int(input("Please enter an integer: ")) if x < 0: x = 0 print('Negative changed to zero') elif x == 0: print('Zero') elif x == 1: print('Single') else: print('More')
Key characteristics:
- TheÂ
elif
 keyword stands for “else if” and helps avoid excessive indentation - You can have zero or moreÂ
elif
 clauses - TheÂ
else
 clause is optional - This structure serves as Python’s alternative to switch/case statements
For more complex pattern matching, consider Python’s match
statement.
Iterating with for
Loops
Python’s for
loop iterates over items in any sequence (lists, strings, etc.) rather than arithmetic progressions:
words = ['cat', 'window', 'defenestrate'] for w in words: print(w, len(w))
When modifying collections during iteration, it’s safer to:
- Iterate over a copy of the collection, or
- Create a new collection
Example:
users = {'Hans': 'active', 'Éléonore': 'inactive', '景太郎': 'active'} active_users = {} for user, status in users.items(): if status == 'active': active_users[user] = status
Generating Number Sequences with range()
For numeric iteration, use the range()
function:
python
Copy
for i in range(5): print(i) # Prints 0 through 4
Features:
- The end point is never included
- Can specify start, stop, and step values
- Memory efficient (doesn’t generate all numbers at once)
- Often used withÂ
len()
 for sequence indexing
Loop Control with break
and continue
break
 exits the innermost loopcontinue
 skips to the next iteration
Example of finding factors:
python
Copy
for n in range(2, 10): for x in range(2, n): if n % x == 0: print(f"{n} equals {x} * {n//x}") break
The else
Clause in Loops
A loop’s else
clause executes when the loop completes normally (without hitting a break
):
for n in range(2, 10): for x in range(2, n): if n % x == 0: break else: print(n, 'is prime')
The Placeholder pass
Statement
pass
does nothing and is used when syntax requires a statement but no action is needed:
class MyEmptyClass: pass def initlog(*args): pass # TODO: Implement this
Pattern Matching with match
Python’s match
statement compares a value against patterns:
def http_error(status): match status: case 400: return "Bad request" case 404: return "Not found" case _: return "Something's wrong"
Advanced features include:
- Combining patterns withÂ
|
- Variable binding in patterns
- Class pattern matching
- Guards withÂ
if
 conditions - Sequence and mapping patterns
Defining Functions
Basic function syntax:
def fib(n): """Print Fibonacci numbers up to n""" a, b = 0, 1 while a < n: print(a, end=' ') a, b = b, a+b print()
Key aspects:
def
 keyword starts the definition- Docstrings provide documentation
- Variables are local by default
- Functions are objects that can be assigned to variables
- Implicitly returnÂ
None
 if no return statement
Advanced Function Features
Default Argument Values
def ask_ok(prompt, retries=4, reminder='Please try again!'): while True: reply = input(prompt) if reply in {'y', 'ye', 'yes'}: return True if reply in {'n', 'no', 'nop', 'nope'}: return False retries -= 1 if retries < 0: raise ValueError('invalid user response') print(reminder)
Important: Default values are evaluated only once at function definition time.
Keyword Arguments
Functions can be called with arguments by name:
def parrot(voltage, state='a stiff', action='voom'): print("-- This parrot wouldn't", action, end=' ') print("if you put", voltage, "volts through it.") print("-- It's", state, "!")
Special Parameter Types
You can define parameters as:
- Positional-only (beforeÂ
/
) - Positional-or-keyword
- Keyword-only (afterÂ
*
)
Example:
def combined_example(pos_only, /, standard, *, kwd_only): print(pos_only, standard, kwd_only)
Arbitrary Argument Lists
Use *args
for variable numbers of positional arguments and **kwargs
for keyword arguments:
python
Copy
def write_multiple_items(file, separator, *args): file.write(separator.join(args))
Lambda Expressions
Small anonymous functions:
pairs = [(1, 'one'), (2, 'two'), (3, 'three')] pairs.sort(key=lambda pair: pair[1])
Documentation Strings
Conventions for docstrings:
- First line: Brief summary
- Second line: Blank
- Following lines: Detailed description
- Maintain consistent indentation
Function Annotations
Optional type hints:
def f(ham: str, eggs: str = 'eggs') -> str: print("Annotations:", f.__annotations__) return ham + ' and ' + eggs
Python Coding Style Guidelines
Key recommendations from PEP 8:
- Use 4 spaces per indentation level
- Limit lines to 79 characters
- Use blank lines to separate logical sections
- Use consistent naming (CamelCase for classes, lowercase_with_underscores for functions)
- Use spaces around operators and after commas
- Use UTF-8 encoding
- Write descriptive docstrings
Following these conventions makes your code more readable and maintainable.